Bit Shift Calculator
The bit shift calculator helps you determine the change in bit operations with decimal, hexadecimal, binary, and octal numbers.
Choose the data type from binary, decimal, or octal to start bit shifting. Choose the bit shifts from 4,8, 12, or a custom number. Choose the direction from left or right. Finally, enter the number of shifts.
The result section shows the binary input, binary result, octal result, unsigned decimal result, and signed decimal result.
In case you don’t know, bit shifts efficiently reorder computer memory bits. Moving bits left or right optimizes low-level programming and minimizes CPU calculations.
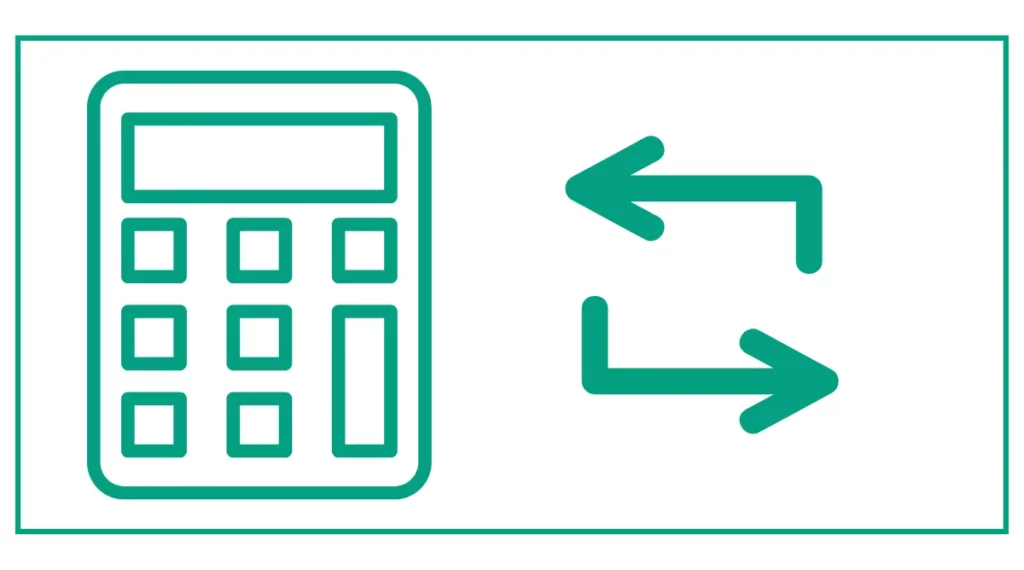
You might want to determine the speed of the audiobook or resonant circuit bandwidth.
A bit shift calculator is an essential tool for performing bitwise operations on binary numbers. This calculator enables users to execute bit shifts, which are fundamental operations in computer programming and digital logic. By understanding and utilizing bit shift operations, programmers and digital enthusiasts can manipulate data at the binary level, leading to more efficient algorithms and optimized code.
What is a Bit Shift Operation?
A bit shift operation moves the digits of a binary number left or right by a specified number of positions. This process can be visualized as sliding the bits of a number in a particular direction, with the outermost bits being discarded and new bits being introduced on the opposite end.
There are two main types of bit shift operations:
- Logical Shift
- Arithmetic Shift
Logical Shift
In a logical shift, the bits are moved left or right, and the empty positions are filled with zeros. This operation is straightforward and does not take into account the sign of the number.
Example of a logical left shift by 2 positions:
Original: 00101100
Shifted: 10110000
Example of a logical right shift by 2 positions:
Original: 00101100
Shifted: 00001011
Arithmetic Shift
An arithmetic shift is similar to a logical shift, but it preserves the sign of the number when shifting right. For signed binary numbers, the leftmost bit (most significant bit) represents the sign. In an arithmetic right shift, this sign bit is copied to fill the empty positions.
Example of an arithmetic right shift by 2 positions:
Original: 10101100 (negative number)
Shifted: 11101011 (sign bit preserved)
How to Use a Bit Shift Calculator
Using a bit shift calculator is generally straightforward. Here’s a step-by-step guide:
- Enter the binary number you want to shift.
- Specify the number of positions to shift.
- Choose the direction of the shift (left or right).
- Select the type of shift (logical or arithmetic).
- Click the “Calculate” or “Shift” button to perform the operation.
The calculator will then display the result of the shift operation, typically in binary and decimal formats.
Applications of Bit Shift Operations
Bit shift operations have numerous applications in computer science and digital electronics. Some common uses include:
- Multiplication and division by powers of 2
- Implementing efficient algorithms
- Encoding and decoding data
- Manipulating individual bits in hardware registers
Multiplication and Division by Powers of 2
One of the most practical applications of bit shifting is performing quick multiplication or division by powers of 2. Left-shifting a binary number by n positions is equivalent to multiplying it by 2^n, while right-shifting by n positions is equivalent to dividing by 2^n (with truncation for odd numbers).
Example:
5 (decimal) = 101 (binary)
Left shift by 2: 10100 (binary) = 20 (decimal)
5 * 2^2 = 5 * 4 = 20
This operation is much faster than traditional multiplication or division, making it valuable in performance-critical applications.
Implementing Efficient Algorithms
Bit shift operations are often used to implement efficient algorithms, particularly in areas like cryptography, compression, and graphics processing. These operations allow for quick manipulation of data at the bit level, which can lead to significant performance improvements.
For example, the XOR (exclusive or) operation, combined with bit shifts, is commonly used in encryption algorithms to scramble data quickly and securely.
Encoding and Decoding Data
Bit shifting is crucial in various encoding and decoding schemes. For instance, when working with color values in computer graphics, bit shifts are used to extract or combine individual color components (red, green, blue) from a single integer representation.
Example:
RGB Color: 0xRRGGBB
Extract Red: (color >> 16) & 0xFF
Extract Green: (color >> 8) & 0xFF
Extract Blue: color & 0xFF
Manipulating Hardware Registers
In embedded systems and low-level programming, bit shift operations are essential for manipulating individual bits in hardware registers. This allows programmers to control specific hardware features or read status information efficiently.
Advanced Features of Bit Shift Calculators
While basic bit shift calculators perform simple left and right shifts, more advanced calculators offer additional features:
- Support for different number systems (binary, decimal, hexadecimal, octal)
- Signed and unsigned integer operations
- Circular shifts (rotation)
- Bitwise AND, OR, XOR, and NOT operations
- Conversion between different number systems
These advanced features make bit shift calculators versatile tools for a wide range of bitwise operations and calculations.
Understanding Binary Representation
To effectively use a bit shift calculator, it’s crucial to understand binary representation. In the binary number system, each digit represents a power of 2, with the rightmost digit representing 2^0, the next 2^1, then 2^2, and so on.
For example, the binary number 1010 represents:
1 * 2^3 + 0 * 2^2 + 1 * 2^1 + 0 * 2^0 = 8 + 0 + 2 + 0 = 10 (decimal)
Understanding this representation helps in interpreting the results of bit shift operations and in predicting how shifts will affect the value of a number.
Bitwise Operations and Bit Shift Calculators
While bit shifting is a fundamental operation, it’s often used in conjunction with other bitwise operations. Many bit shift calculators also support these additional operations:
- AND (&): Sets each bit to 1 if both bits are 1
- OR (|): Sets each bit to 1 if at least one bit is 1
- XOR (^): Sets each bit to 1 if only one of the bits is 1
- NOT (~): Inverts all the bits
These operations, combined with bit shifting, form the basis of many low-level algorithms and data manipulations.
Practical Examples Using a Bit Shift Calculator
Let’s explore some practical examples of using a bit shift calculator:
Example 1: Multiplying by 4
To multiply a number by 4 using bit shifts, we can shift left by 2 positions:
Input: 5 (binary 101)
Left shift by 2: 10100
Result: 20 (5 * 4)
Example 2: Dividing by 2
To divide a number by 2, we can use a right shift by 1 position:
Input: 14 (binary 1110)
Right shift by 1: 111
Result: 7 (14 / 2)
Example 3: Extracting a nibble
To extract the lower nibble (4 bits) from a byte:
Input: 10110011
Right shift by 4: 00001011
AND with 1111: 00001011
Result: 1011 (11 in decimal)
These examples demonstrate how bit shift operations can be used to perform various calculations and data manipulations efficiently.
Limitations and Considerations
While bit shift calculators are powerful tools, it’s important to be aware of their limitations:
- Overflow: Shifting left can cause overflow if the result exceeds the maximum value representable in the given number of bits.
- Precision: Right shifts on signed numbers may lead to loss of precision due to truncation.
- Language-specific behavior: Different programming languages may handle bit shifts slightly differently, especially for negative numbers or shifts larger than the word size.
Conclusion
Bit shift calculators are invaluable tools for anyone working with binary operations, from computer science students to professional programmers and hardware engineers. By understanding the principles of bit shifting and using these calculators effectively, you can perform efficient calculations, optimize algorithms, and gain a deeper understanding of how computers manipulate data at the most fundamental level.
References
- Bitwise left and right shift operators >. (n.d.). Bitwise Left and Right Shift Operators >. ibm.com/docs/ssw_ibm_i_72/rzarg/bitshe.htm
- T. (2023, March 13). Left shift and right shift operators (‘>’). Left Shift and Right Shift Operators (‘<>’) | Microsoft Learn. learn.microsoft.com/en-us/cpp/cpp/left-shift-and-right-shift-operators-input-and-output?view=msvc-170
- Bit Shifting. (n.d.). Bit Shifting. inst.eecs.berkeley.edu/~cs61bl/r/cur/bits/bit-shifting.html